How to get all the transactions of an address on Solana in JavaScript
In this tutorial, we are going to learn how to get all the transactions of an address on the Solana network with JavaScript and the Solana Web3.js library or the JSON-RPC API.
In this tutorial, we are going to learn how to get all the transactions of an address on the Solana network with JavaScript and the Solana Web3.js library or the JSON-RPC API.
Get the transactions using the Solana Web3.js library
To get all the transaction of an address, we have 2 options, either we use the Solana Web3.js or the Solana JSON-RPC API.
To do both, first install the Solana Web3.js library:
npm install @solana/web3.js
And then use it to get a URL to interact with the blockchain and create a connection to the network you want (the mainnet, the devnet or the testnet):
import { clusterApiUrl, Connection } from '@solana/web3.js'
// you can also pass 'testnet' or 'devnet' here
const URL = clusterApiUrl('mainnet-beta');
const connection = new Connection(URL)
If you're using React, you can just use the useConnection
hook instead of creating a new connection to the blockchain.
And then you can call the getSignaturesForAddress
function which will return the list of signatures of all the transaction that an address has sent on the blockchain.
import { PublicKey } from '@solana/web3.js'
const address = new PublicKey("GX6nkQgcXy4xDyuSH9MKjG9nq5KN5ntE3ZUUHSqUrcC8")
const signatures = await connection.getSignaturesForAddress(address)
That function takes in parameter the address you want to get the signatures of, as a PublicKey
object, and it returns an array of ConfirmedSignatureInfo
objects with a signature
property.
If you're using React, you can use the useWallet
hook to get the PublicKey
of the connected wallet directly.
Then, using these signatures, you can fetch information about each transaction using the getParsedTransaction
function. If you want want to get more information about all the transactions at once, use the getParsedTransactions
function.
Here is an example using the getParsedTransactions
function:
import { PublicKey } from '@solana/web3.js'
const address = new PublicKey("GX6nkQgcXy4xDyuSH9MKjG9nq5KN5ntE3ZUUHSqUrcC8")
const signaturesRes = await connection.getSignaturesForAddress(address)
const signatures = signaturesRes.map(tx => tx.signature)
const data = await connection.getParsedTransactions(signatures)
Or with the getParsedTransaction
function:
import { PublicKey } from '@solana/web3.js'
const address = new PublicKey("GX6nkQgcXy4xDyuSH9MKjG9nq5KN5ntE3ZUUHSqUrcC8")
const signaturesRes = await connection.getSignaturesForAddress(address)
const signatures = signaturesRes.map(tx => tx.signature)
const data = await connection.getParsedTransaction(signatures[0])
Both functions will return the same type of data. The getParsedTransactions
function will return an array of ParsedTransactionWithMeta
and the getParsedTransaction
function will return a single ParsedTransactionWithMeta
.
Check out the documentation to learn more about each function and the response objects:
- The
getSignaturesForAddress
function - The
ConfirmedSignatureInfo
object that is in the array returned by thegetSignaturesForAddress
- The
getParsedTransaction
function - The
getParsedTransactions
function - The
ParsedTransactionWithMeta
object returned by both thegetTransaction
andgetTransactions
functions
Get the transactions using the JSON-RPC API
Here is an example in which I get the signatures of all the transactions of an address on the Mainnet:
import solanaWeb3 from '@solana/web3.js';
const URL = solanaWeb3.clusterApiUrl('mainnet-beta'); // you can also pass 'testnet' or 'devnet' here
const res = await fetch(URL, {
method: 'POST',
headers: { 'content-type': 'application/json' },
body: JSON.stringify({
jsonrpc: '2.0',
id: 'get-signatures',
method: 'getSignaturesForAddress',
params: [address],
}),
});
const jsonRes = await res.json();
const signatures = jsonRes.result.map(result => result.signature)
In the code above, we send an API call to the blockchain URL we got from above. It's a POST
request and in the body
, we pass information about the data we want to get.
The way JSON-RPC APIs work is they have methods that you can call and send specific parameters.
In this case, we want to call the method called getSignaturesForAddress
so that's what we pass in the method
property in the body of the request.
That method expects an address in parameters so in the params
property we pass the address of the wallet we want to get the transactions of.
So in the code above, address
is a string containing the address that you want to get the transaction signatures for.
The id
property in the body is just an identifier that will be in the response of the API call and that helps you identify the request and the response.
In the response of that API call, there is a result
property that contains all the signatures of all the transactions ever made by the address you passed in the parameters.
Here is an example of result you'll get from that API call:
{
"jsonrpc": "2.0",
"result": [
{
"err": null,
"memo": null,
"signature": "5h6xBEauJ3PK6SWCZ1PGjBvj8vDdWG3KpwATGy1ARAXFSDwt8GFXM7W5Ncn16wmqokgpiKRLuS83KUxyZyv2sUYv",
"slot": 114,
"blockTime": null
}
],
"id": 1
}
In this example, there is only one signature but you'll have multiple objects like this in the result
if the address has sent multiple transactions or an empty array if the address has never sent a transaction.
Then, when you have a list of all the transaction signatures of an address, you can use that list to get the details of each transaction using the following API call:
const signature = 'the transaction signature here'
const res = await fetch(URL, {
method: 'POST',
headers: { 'content-type': 'application/json' },
body: JSON.stringify({
jsonrpc: '2.0',
id: 'get-transaction',
method: 'getTransaction',
params: [signature],
}),
});
const transaction = await res.json();
So here the API call is similar to the one above, but the method we call is getTransaction
and in the parameters we pass the signature of the transaction we want to get information for.
The object returned in the result
property is more complicated than before so you can take a look at the documentation to see exactly all the properties available: https://docs.solana.com/developing/clients/jsonrpc-api#gettransaction
Also, check out our guide on how to get a Solana transaction with JavaScript, it will guide you step-by-step and give you more insights about the result object and how to get the data that you want from it.
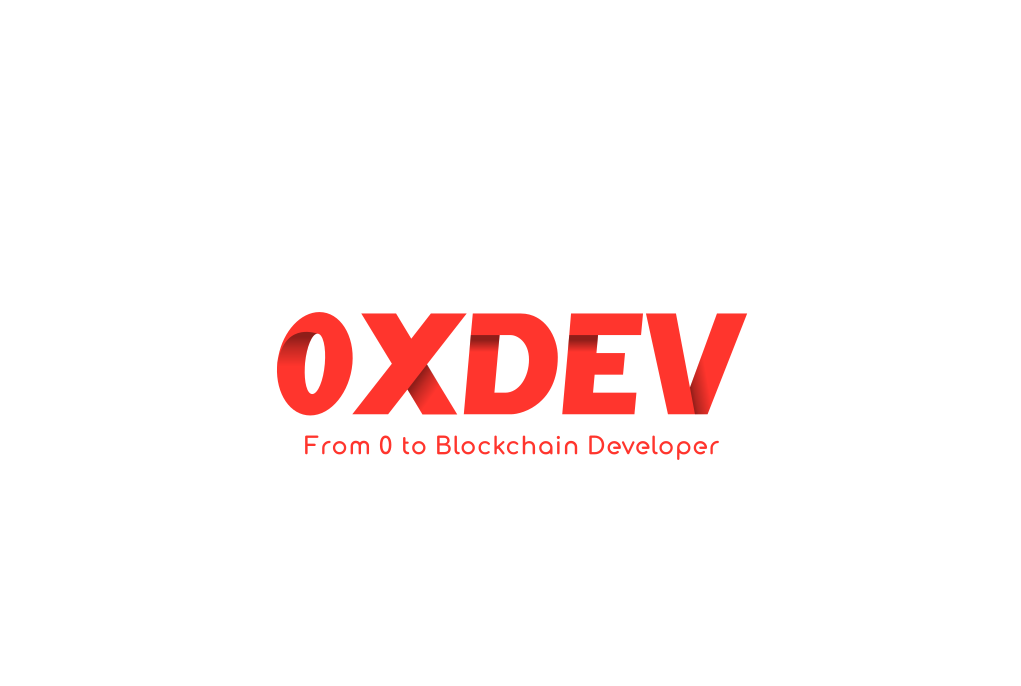
Don't use the public URLs in production
Note that using the public blockchain URLs that the clusterApiUrl
provides can lead to issues because these URLs have rate-limiting so if you send too many API calls at a time, it will return an error at some point.
You shouldn't use these URLs in production. Instead, you can use a blockchain API provider that supports the Solana blockchain like GetBlock, Alchemy or QuickNode.
These services manage nodes on the Solana blockchain and they will give you URLs that you can use to interact with the blockchain through that node. Basically, you send the same API calls to these URLs and they forward them to the blockchain.
Otherwise, you can create and manage your own node but it's way more complex.
And that's it 🎉
Thank you for reading this article