How to send ERC-20 tokens to another address in React using Wagmi
In this tutorial, we are going to learn how to send ERC-20 tokens from the wallet connected to your React app to another address using Wagmi.
In this tutorial, we are going to learn how to send ERC-20 tokens from the wallet connected to your React app to another address using Wagmi.
To do that, we can use the usePrepareContractWrite
and the useContractWrite
hooks:
import { useContractWrite, usePrepareContractWrite } from 'wagmi'
import { utils } from 'ethers'
// Send 100 LINK tokens
function SendTokens() {
// prepare the transaction
const { config } = usePrepareContractWrite({
address: "0x514910771AF9Ca656af840dff83E8264EcF986CA",
abi: [{
"constant": false,
"inputs": [
{"name":"_to","type":"address"},
{"name":"_value","type":"uint256"}
],
"name":"transfer",
"outputs": [{"name":"success","type":"bool"}],
"stateMutability": "nonpayable",
"type": "function"
}],
functionName: "transfer",
args: [
"0x5F70Ddd9908B04f952b9cB2A6F8E4D451725ceDC",
utils.parseEther("100") // convert to wei
]
})
// get the transfer function
const {
data,
isLoading,
isSuccess,
write
} = useContractWrite(config)
if (isLoading) {
return <p>Waiting for confirmations on your wallet...</p>
}
if (isSuccess) {
return <p>Transaction was sent! Waiting for it to complete</p>
}
return (
<div>
<button disabled={!write} onClick={() => write?.()}>
Send 100 LINK
</button>
</div>
)
}
In the example above, we send 100 LINK tokens to the address passed to args
property in usePrepareContractWrite
from the wallet the user connected to your app.
To do that, we use the usePrepareContractWrite
hook with the following arguments:
address
: the address of the smart contract of the token to sendabi
: the ABI of the smart contract containing at least thetransfer
functionfunctionName
: the name of the function in the smart contract that you want to call. In this case it's thetransfer
functionargs
: the arguments to pass to the function you are calling. As described in the ABI, thetransfer
function takes in 2 parameters, first the address we want to send the tokens to and second, the amount of tokens to send
These are the arguments that you need to pass but you can pass other arguments to send the transaction a bit differently like with a specific gas limit for example.
Check out the documentation to see the full list of arguments you can pass here.
The usePrepareContractWrite
hook will return a config object that you can pass to the useContractWrite
hook which will return a write
function (among other values) that you can call to send the transaction.
Again, passing the config is required but you can pass it a bit differently and pass other arguments to the useContractWrite
hook.
Check out the documentation to see all the arguments you can pass.
That hook will return the following values (among others, but these are the ones that we use the most):
write
: a function you can call to fire the transaction that calls the function in the smart contractdata
: an object containing the transaction data:
•hash
: the hash of the transaction
•wait
: a function you can call to wait for the transaction to complete (takes in parameter the number of confirmations and returns a TransactionReceipt or throws an error)error
: if the transaction could not be sent, it will contain an error object with information about what went wrong. Otherwise, it'snull
.
There will also be an error if the user rejects the transaction on their wallet.isLoading
:true
after callingwrite
while waiting for the user to confirm the transaction on their wallet andfalse
otherwiseisError
:true
when sending the transaction failed andfalse
otherwise. It will also be true if the users reject the transaction on their wallet.isSuccess
:true
if the transaction was sent successfully andfalse
otherwise. It will also befalse
before sending the transaction and while waiting for the user's confirmation.
Check out the documentation here to see the list of return values.
Now, after the transaction is sent, it's still pending, it hasn't been confirmed on the blockchain yet. If you want to wait for the transaction to complete and inform the user when it's done, you can use the wait
function inside the data
returned by useContractWrite
or use the useWaitForTransaction
hook.
Check out this tutorial to learn how to wait for a transaction to complete using Wagmi:
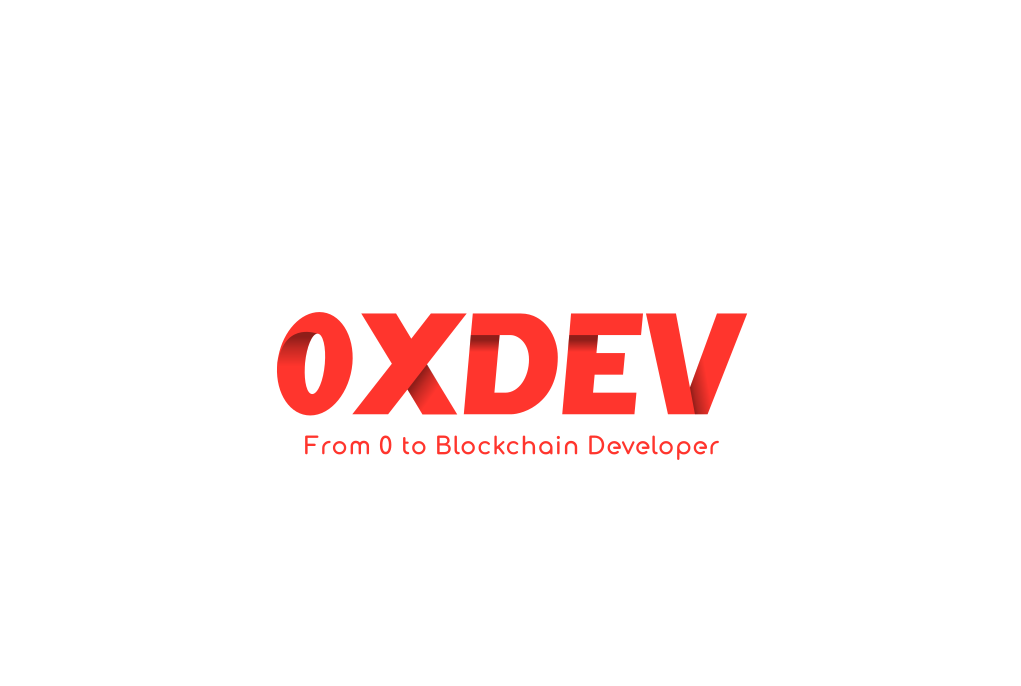
And that's it 🎉
Thank you for reading this article