The complete guide to use Wagmi hooks in a React app
Learn how to configure Wagmi in your React application and how to use the Wagmi hooks to interact with connected wallets, contracts and the blockchain.
In this tutorial, we are going to learn how to configure Wagmi in your React application and how to use the Wagmi hooks to interact with connected wallets, contracts and the blockchain.
Here is an example of how to set up a Wagmi client to be able to use the hooks:
import { chain, configureChains, createClient, WagmiConfig } from 'wagmi';
// connectors
import { InjectedConnector } from 'wagmi/connectors/injected';
import { CoinbaseWalletConnector } from 'wagmi/connectors/coinbaseWallet';
import { MetaMaskConnector } from 'wagmi/connectors/metaMask';
import { WalletConnectConnector } from 'wagmi/connectors/walletConnect';
// providers
import { infuraProvider } from 'wagmi/providers/infura';
import { alchemyProvider } from 'wagmi/providers/alchemy';
import { publicProvider } from 'wagmi/providers/public';
// Define the chains we want to interact with and the providers we will use
const { chains, provider } = configureChains(
// The chains you want to use
[chain.mainnet],
// The providers to use for these chains
[
infuraProvider({ apiKey: 'API_KEY' }),
alchemyProvider({ apiKey: 'API_KEY' }),
publicProvider()
],
);
// Define the wallets that we want to allow the users to connect to the app
const connectors = [
new InjectedConnector(),
new CoinbaseWalletConnector({
options: {
appName: '0xDev Test',
},
}),
new MetaMaskConnector(),
new WalletConnectConnector({
options: {
qrcode: true,
},
}),
];
// Create a Wagmi client
const wagmiClient = createClient({
autoConnect: false,
connectors,
provider,
});
// Wrap the app in a WagmiConfig and pass in the client
function App() {
return (
<WagmiConfig client={wagmiClient}>
<div className="App">
</div>
</WagmiConfig>
);
}
export default App;
As you can see, the steps to set up and use Wagmi are:
- Configure the chain(s) that your app will use
- Configure providers that allow your app to talk to the blockchains you want (Infura, Alchemy, a custom RPC client or the public provider)
- Configure the connectors which are the wallets that you want to allow users to connect to your app
- Using the chains, providers and connectors, create a Wagmi client
- Wrap your app in a
WagmiConfig
and pass the Wagmi client in the props
And now, the components that are rendered inside the WagmiConfig
can use any Wagmi hook.
Since you wrapped the whole app in a WagmiConfig
, all the components can start using Wagmi hooks!
Configuring the chains and providers
To configure the chains that you want your app to use, we use the configureChains
function.
That function returns the chains
that we set our app to use and the provider
that our app will use to communicate with the blockchain. We can then use that provider to create the Wagmi client.
The configureChains
function takes parameter 2 arrays:
First, the list of chains to use. In that array, you can pass any value that is in the chain
object that you can import from Wagmi like this:
import { chain } from 'wagmi'
To see the full list of chains that this object contains, check out this file in Wagmi's Github repository.
Second, the list of providers to use to interact with the chains you passed. You can use of of these providers:
infuraProvider
: the Infura blockchain API. You have to pass an API key in the parameters of that provider.alchemyProvider
: the Alchemy blockchain API. Just like Infura, you need to pass an API key in the parameters of that provider.publicProvider
: the public API URLs of each blockchain that allow you to interact with them.
Don't use it in production as these URLs have rate-limiting.
Otherwise your app might throw unexpected errors.jsonRpcProvider
: a custom RPC URL. Learn more about this specific provider here: https://wagmi.sh/docs/providers/jsonRpc
If you pass multiple providers, it will use the first one unless it doesn't support the network that your app is using. In that case, it will try using the other providers.
Here is an example of how to use the configureChains
function:
import { configureChains, createClient, chain } from 'wagmi'
import { infuraProvider } from 'wagmi/providers/infura'
import { publicProvider } from 'wagmi/providers/public'
const { chains, provider } = configureChains(
[chain.mainnet], // The main Ethereum network
[
infuraProvider({ apiKey: 'YOUR_INFURA_API_KEY' }),
publicProvider(),
]
)
const wagmiClient = createClient({
autoConnect: true,
connectors: [
// Your connectors here
],
provider,
})
Configuring the connectors
The connectors are the wallets that you want your users to be able to connect to your app. It's the wallets that the Wagmi client supports.
To create a Wagmi client, you must create an array of connectors. Otherwise users can't connect their wallet to your app.
Wagmi provides 4 connectors:
InjectedConnector
: Allows to connect any wallet with a browser extension like MetaMask.CoinbaseWalletConnector
: Displays a modal that allows to connect a Coinbase wallet, either through the mobile app or the browser extensionMetaMaskConnector
: Allows to connect a MetaMask wallet through the mobile app or the browser extensionWalletConnectConnector
: Displays a modal with a QR code that allows to connect a wallet through WalletConnect.
Check out the documentation to learn more about each connector.
Here is an example of creating an array of connectors with all the connectors that Wagmi provides:
import { InjectedConnector } from 'wagmi/connectors/injected';
import { CoinbaseWalletConnector } from 'wagmi/connectors/coinbaseWallet';
import { MetaMaskConnector } from 'wagmi/connectors/metaMask';
import { WalletConnectConnector } from 'wagmi/connectors/walletConnect';
const connectors = [
new InjectedConnector(),
new CoinbaseWalletConnector({
options: {
appName: '0xDev Test',
},
}),
new MetaMaskConnector(),
new WalletConnectConnector({
options: {
qrcode: true,
},
}),
];
Stick to the end of this tutorial, we will discuss how to connect a wallet more in details.
Once you have your array of connectors and the provider, you can create the Wagmi client
Creating the Wagmi Client
Now that we have our provider from configuring the chains and the providers and that we have our connectors, we can create a Wagmi Client. false
To do that, we use the createClient
function. That function takes in parameter an object in which you can pass the following properties:
autoConnect
(optional): A boolean (false
by default) indicating if you want to automatically re-connect the user's wallet when they come back to your app.connectors
(optional): The list of connectors your app uses (see the connectors secion of this tutorial)logger
(optional): A custom function to call when Wagmi tries to log things. By default it uses theconsole
logs.provider
(required): The Ethers JS provider to use to interact with the blockchain you want (see the configuring chains section)storage
(optional): How and where to persist Wagmi's cache data. By default, it stores the cache inwindow.localStorage
.webSocketProvider
(optional): An Ethers JS Websocket provider to interact with the blockchain using WebSockets.
Note that passing connectors is optional but if you don't do it, you won't be able to allow users to connect a wallet to your app.
The createClient
function will return a Wagmi client that you then need to pass in the props of the WagmiConfig
component and that component should wrap your whole app so we usually put it in the App.js
file.
Here is an example:
import { chain, configureChains, createClient, WagmiConfig } from 'wagmi';
// connectors
import { InjectedConnector } from 'wagmi/connectors/injected';
// providers
import { infuraProvider } from 'wagmi/providers/infura';
const { provider } = configureChains(
[chain.mainnet],
[infuraProvider({ apiKey: 'API_KEY' })],
);
// Create a Wagmi client
const wagmiClient = createClient({
autoConnect: false,
connectors: [new InjectedConnector()],
provider,
});
function App() {
return (
<WagmiConfig client={wagmiClient}>
<div className="App">
</div>
</WagmiConfig>
);
}
export default App;
Check out this section in the documentation to learn more about this function.
How to use the Wagmi hooks
Now that you wrapped your whole app in a WagmiConfig
component and passed a Wagmi client to it, you can use any Wagmi hook available.
The Wagmi hooks all work following a common pattern
- They take in parameter an object in which you can configure how you want to use the hook. Of course, there are exceptions, a few hooks don't take anything in parameters.
- They return an object containing the data you're looking for and information about the execution of the hook. Again, there are exceptions and some hooks directly return the data you want, not in an object.
The majority of the hooks return an object containing at least the following properties:
data
: The data that was fetched or the result of the transaction that was sent or the result of the action you executed.error
: If fetching data or executing the action you wanted to execute failed, that property will contain the error that was thrown.
An example of error that this property can contain is if the user rejected to do what you wanted them to do on their wallet.isLoading
: A boolean that will betrue
while fetching data or waiting for the user to confirm executing the action you want to do with their wallet.
Every hook is different but that's the pattern that Wagmi uses. Once you've used a few hooks, you'll get used to it and you'll see the similarities and it will make a lot more sense.
If you want to learn how to do specific things with Wagmi, you can check out our whole list of tutorials about Wagmi here.
The next step after this tutorial would be to learn how to connect a wallet to your app (see the section below) and then to learn how to interact with a smart contract using Wagmi.
Connecting a wallet to your app
Now, you need to have a way for your users to connect their wallet to your app. At this point, we've just told Wagmi what connectors we want to use in order to support specific wallets.
We need to create a button that allows the users to connect their wallet. It's a completely separate topic so we created separate tutorials on how to do it using different tools and approaches.
So to learn how to connect a wallet to your React app that uses Wagmi, check out these tutorials (I highly recommend using either RainbowKit or Web3Modal as the appearance and experience will be way better):
- Connect a wallet using the default Wagmi look and experience:
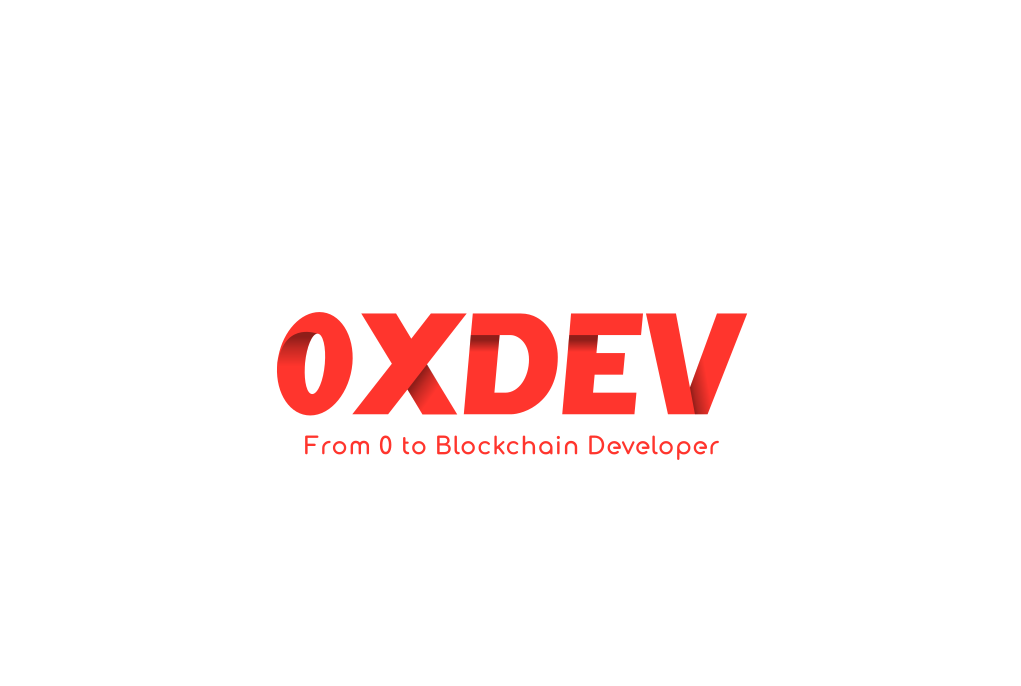
- Connect a wallet with RainbowKit (which does the same but displays a pretty modal):
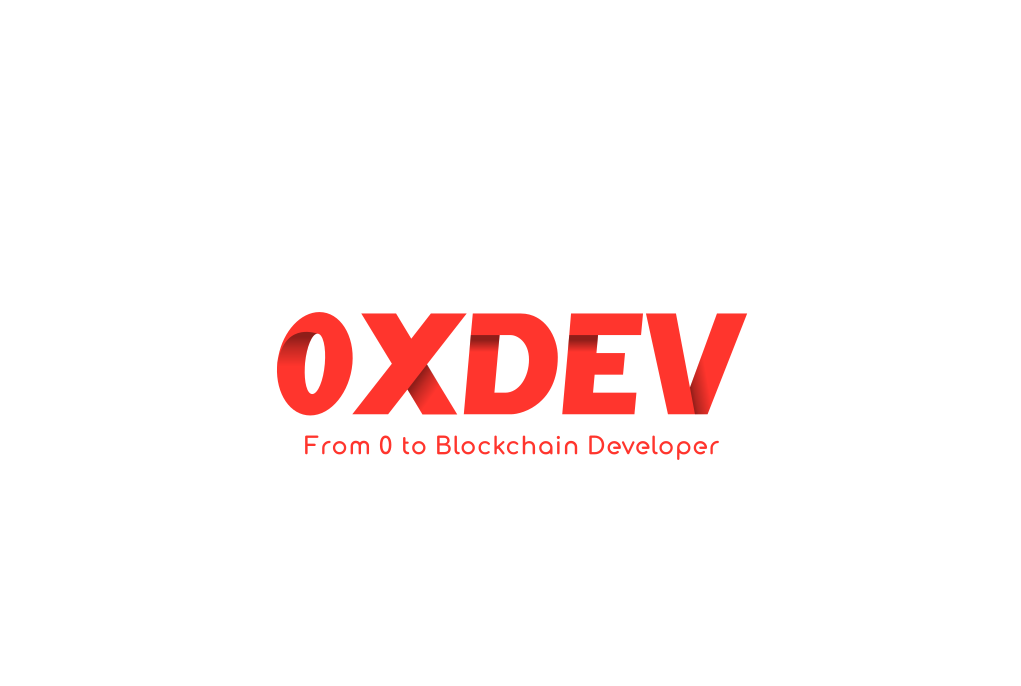
- Connect a wallet using Web3Modal (which also displays a pretty modal):
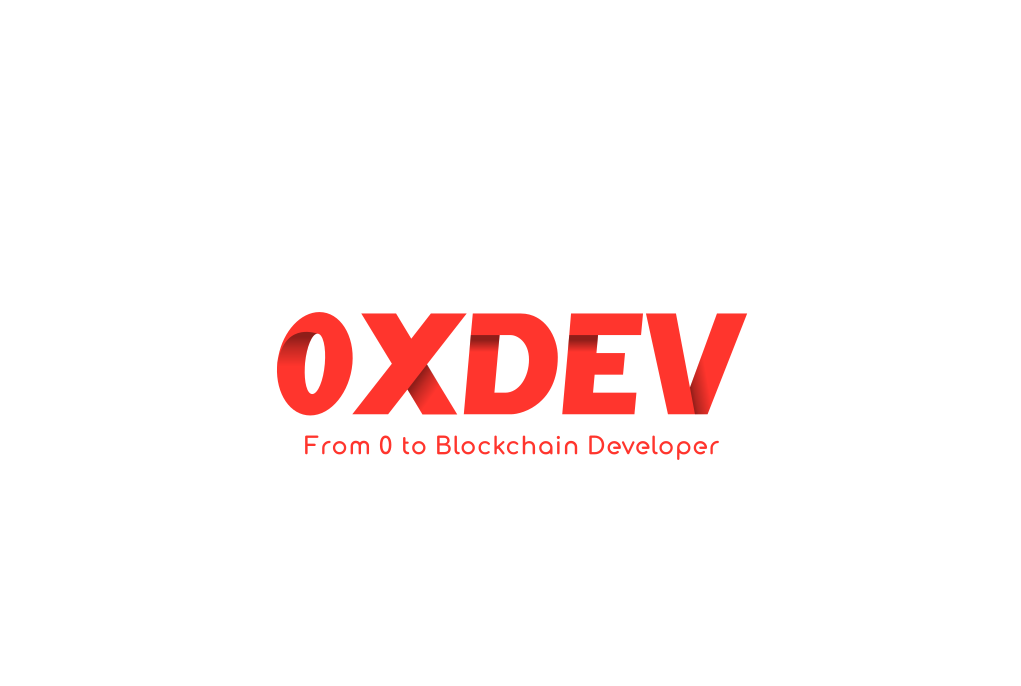
And that's it 🎉
Thank you for reading this article